728x90
HttpServlet을 상속받아서 요청/응답 처리를 해주는 서블릿을 구현할것이다.
주소 : localhost8282/helloServlet?username="kim"
1. 서블릿 등록하기
package hello.servlet.basic;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
@WebServlet(name="helloServlet", urlPatterns = "/hello")
public class HelloServlet extends HttpServlet {
@Override
//요청이 오면 request를 서블릿에 던져줌. 응답을 위한 response 객체를 넘겨준다
protected void service(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
System.out.println("HelloServlet.service"); //ctr+o 오버라이드 메서드
System.out.println("request=" + request); //soutm
System.out.println("response=" + response);
//서블릿은 쿼리파라미터를 편하게 읽도록 지원을 해줌
//localhost:8282/hello?username=kim;
String username = request.getParameter("username");
System.out.println("username = " + username);
//응답할때 정보를 보내는것 (set 해주는것)
// 단순문자로 보낸다 : text/plain
response.setContentType("text/plain");
response.setCharacterEncoding("utf-8");
//http body에 메세지가 들어감
response.getWriter().write("hello" + username);
}
}
★ 코드분석 ★
- @WebServlet(name="helloServlet", urlPatterns = "/hello")
@WebServlet 서블릿 애노테이션
- name : 서블릿 이름
- urlPatterns : URL 매핑
- protected void service(HttpServletRequest request, HttpServletResponse response)
HTTP 요청을 통해 매핑된 URL이 호출되면서 서블릿컨테이너는 이 메서드를 실행한다
- 웹브라우저 실행
http://localhost:8383/hello?username=world
결과 : hello world
2. 서블릿 컨테이너 동작방식
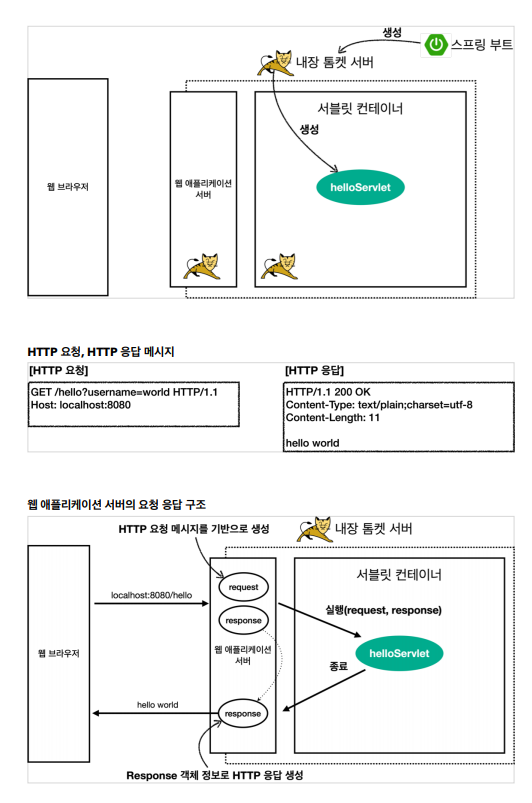
중요 >>> helloServlet은 싱글톤객체를 유지한다!!!!!!!
3. welcome 페이지 추가
경로 : main/webapp/index.html
webapp 경로에 index.html 을 두면 http://localhost:8080 호출시 index.html 페이지가 열린다
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<ul>
<li><a href="basic.html">서블릿 basic</a></li>
</ul>
</body>
</html>
4. basic.html 추가
경로 : main/webapp/basic.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<ul>
<li>hello 서블릿 <ul>
<li><a href="/hello?username=servlet">hello 서블릿 호출</a></li>
</ul>
</li>
<li>HttpServletRequest
<ul>
<li><a href="/request-header">기본 사용법, Header 조회</a></li>
<li>HTTP 요청 메시지 바디 조회
<ul>
<li><a href="/request-param?username=hello&age=20">GET -
쿼리 파라미터</a></li>
<li><a href="/basic/hello-form.html">POST - HTML Form</a></
li>
<li>HTTP API - MessageBody -> Postman 테스트</li>
</ul>
</li>
</ul>
</li>
<li>HttpServletResponse
<ul>
<li><a href="/response-header">기본 사용법, Header 조회</a></li>
<li>HTTP 요청 메시지 바디 조회
<ul>
<li><a href="/response-html">HTML 응답</a></li>
<li><a href="/response-json">HTTP API JSON 응답</a></li>
</ul>
</li>
</ul>
</li>
</ul>
</body>
</html>
5. 실행화면 >>
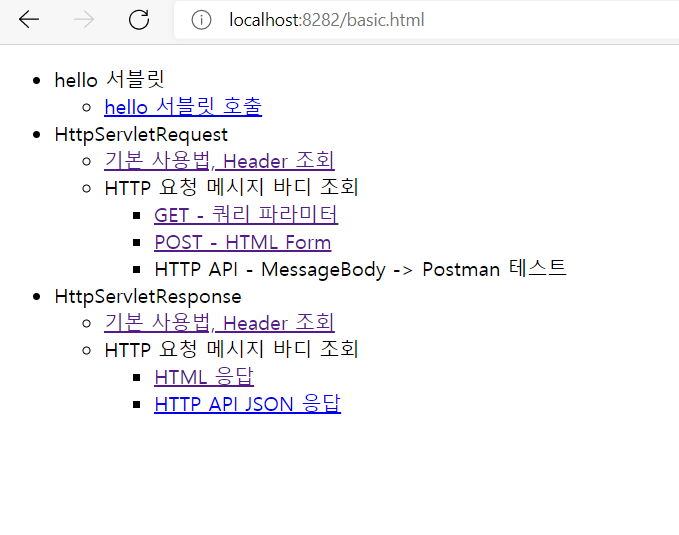
'SPRING > 인프런강의) 스프링 MVC 1편 - 백엔드 웹 개발 핵심 기술' 카테고리의 다른 글
[spring] servlet(1)_프로젝트 생성 (0) | 2021.06.07 |
---|